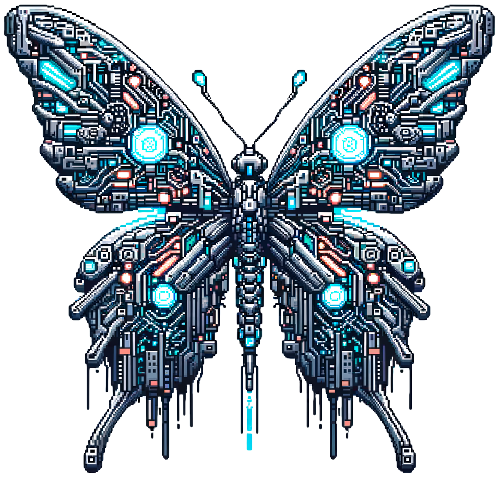
raku.gg
Heredocs in Raku
Hey there, Raku enthusiasts! Today, we’re diving into the wonderful world of heredocs. Now, I know what you’re thinking: “Heredocs? Sounds like something from a medieval fantasy novel!” Well, you’re not entirely wrong – heredocs are indeed magical, but in the realm of programming! 😄
What’s a Heredoc, Anyway?
Imagine you’re writing a long, multi-line string in your code. You could use a bunch of quotation marks and line breaks, but that gets messy fast. Enter the heredoc – your multi-line string superhero! 🦸♀️
A heredoc in Raku is a way to write multi-line strings without all the fuss. It’s like giving your string its own cozy little house in your code. Let’s see how it works!
The Basics: Your First Heredoc
Here’s a simple heredoc in action:
say q:to/END/;
Roses are red,
Violets are blue,
Heredocs are awesome,
And so are you!
END
When you run this, you’ll see:
Roses are red,
Violets are blue,
Heredocs are awesome,
And so are you!
How cool is that? We just wrote a multi-line string without any quotation marks or concatenation. The q:to/END/ tells Raku, “Hey, start a heredoc here and keep going until you see a line that just says ‘END’.”
Getting Fancy: Interpolation with qq
Now, let’s say you want to use variables in your heredoc. That’s where qq comes in handy. It’s like the cool cousin of q that allows interpolation. Check this out:
my $name = "Alice";
my $age = 28;
say qq:to/BIO/;
Name: $name
Age: $age
Hobbies: Coding in Raku,
Drinking coffee,
Explaining heredocs to friends
BIO
This will output:
Name: Alice
Age: 28
Hobbies: Coding in Raku,
Drinking coffee,
Explaining heredocs to friends
Pro tip: Be careful with $ signs in your qq heredocs. If you have a $ that’s not meant to be a variable, you’ll need to escape it with a backslash (\$).
The Indentation Magic Trick 🎩✨
Here’s a neat feature: Raku is smart about indentation in heredocs. If your ending delimiter is indented, Raku will remove that same amount of indentation from every line. It’s like your code is doing a little dance to align everything nicely!
say q:to/SPELL/;
Double, double toil and trouble;
Fire burn and caldron bubble.
Fillet of a fenny snake,
In the caldron boil and bake;
SPELL
Even though we indented the heredoc content, the output will be flush left:
Double, double toil and trouble;
Fire burn and caldron bubble.
Fillet of a fenny snake,
In the caldron boil and bake;
Heredocs in the Wild: Real-world Examples
Let’s look at some fun, practical examples of where heredocs shine:
1. Writing HTML Templates
my $page_title = "My Awesome Raku Blog";
my $content = "Heredocs rock!";
say qq:to/HTML/;
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>$page_title</title>
</head>
<body>
<h1>$page_title</h1>
<p>$content</p>
</body>
</html>
HTML
2. Crafting a Silly Mad Libs Game
my $noun = prompt "Give me a noun: ";
my $verb = prompt "Now a verb: ";
my $adjective = prompt "Finally, an adjective: ";
say qq:to/MADLIB/;
Once upon a time, there was a $adjective $noun.
This $noun loved to $verb all day long.
The end. (Hey, I never said it was a good story!)
MADLIB
3. Creating a Multi-line Regex Pattern
my $regex = rx:to/PATTERN/;
^ # Start of string
\d+ # One or more digits
\s* # Optional whitespace
[:alpha:]+ # One or more letters
# End of string
$
PATTERN
say "42 answer" ~~ $regex; # True
say "Not a match" ~~ $regex; # False
Wrapping Up
Heredocs are like the Swiss Army knife of string literals in Raku. They’re flexible, readable, and can make your code look cleaner than a whistle. 🎶
Remember:
- Use q:to/END/ for literal strings
- Use qq:to/END/ when you need interpolation
- Use qx:to/END/ for regular expression patterns.
- The ending delimiter can be anything you want (not just END)
- Indentation is magically handled for you
Next time you find yourself wrestling with a multi-line string, remember your new superhero friend: the heredoc!
Happy coding, Raku rockstars! 🎸✨
P.S. I once spent an hour debugging a heredoc only to realize I had misspelled the ending delimiter. Don’t be like me – double-check those delimiters! 😅
WWW: 🐪 perl.gg - 🧙♂️ clc.onl - 💾 GitHub - 🏙️ Neocities
Copyright ©️ 2024 raku.gg