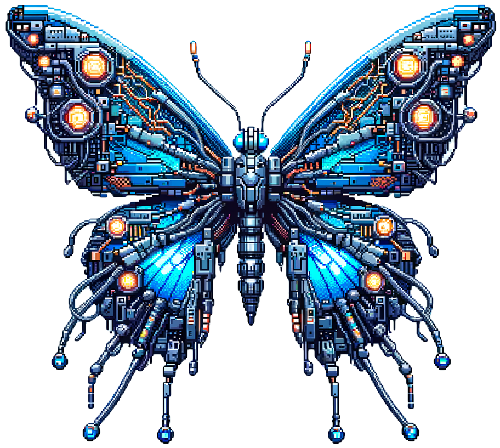
raku.gg
Bridging Two Worlds: Using Inline::Perl5 in Raku
Welcome to the inaugural post of our Raku adventure blog! 🎉 Today, we’re diving straight into the deep end with Inline::Perl5, a game-changing module for Perl 5 veterans venturing into Raku. You might wonder why we’re not starting with the basics, but there’s method to this madness. Inline::Perl5 is like a universal translator between Perl 5 and Raku, allowing you to use your favorite Perl 5 modules in Raku, gradually migrate large codebases, and leverage your existing knowledge while learning Raku. It’s a powerful tool that bridges two worlds, making your transition smoother than a cat’s purr.
What is Inline::Perl5?
As someone who cut their teeth on Perl 5, I know the struggle of wanting to embrace the shiny new features of Raku while still leveraging years of Perl 5 experience and code. That’s where Inline::Perl5 comes in handy. It allows you to:
- Use Perl 5 modules directly in your Raku code
- Gradually migrate large Perl 5 codebases to Raku
- Take advantage of CPAN’s vast module ecosystem
Installing Inline::Perl5
Before we dive in, let’s get Inline::Perl5 installed. The easiest way is to use our trusty package manager, zef:
zef install Inline::Perl5
If you’re feeling adventurous or need a specific version, you can also install it directly from its GitHub repository:
zef install https://github.com/niner/Inline-Perl5.git
Using Inline::Perl5: The Basics
Let’s start with a simple example. Say we want to use the Perl 5
module Data::Dumper
in our Raku code:
use Inline::Perl5;
my $p5 = Inline::Perl5.new;
$p5.use('Data::Dumper');
my %data = (
=> 'Whiskers',
name => 3,
age => ['mouse', 'ball', 'laser pointer']
favorite_toys );
say $p5.call('Dumper', %data);
Let’s break this down:
- We import Inline::Perl5 and create a new instance.
- We use the use method to load the Perl 5 module Data::Dumper.
- We create a Raku hash with some cat data.
- We call the Perl 5 Dumper function on our Raku data structure.
Mixing Perl 5 and Raku Code
One of the coolest features of Inline::Perl5 is the ability to embed Perl 5 code directly in your Raku script. Here’s how:
use Inline::Perl5;
my $p5 = Inline::Perl5.new;
$p5.run(q:to/END/);
sub greet_cat {
my $name = shift;
return "Meow, $name! Welcome to the world of Perl 5 cats.";
}
END
my $name = 'Whiskers';
say $p5.call('greet_cat', $name);
In this example, we define a Perl 5 subroutine greet_cat using the run method, then call it from our Raku code. It’s like teaching your Raku cat to speak Perl 5!
Pro Tip: When working with larger Perl 5 codebases, you can even load entire Perl 5 files using the run_file method:
$p5.run_file('path/to/your/perl5/cat_scripts.pl');
Handling Perl 5 Objects in Raku
Inline::Perl5 doesn’t just stop at functions; it allows you to work with Perl 5 objects too! Let’s create a simple Perl 5 Cat class and see how we can use it in Raku:
use Inline::Perl5;
my $p5 = Inline::Perl5.new;
$p5.run(q:to/END/);
package Cat;
sub new {
my ($class, $name) = @_;
return bless { name => $name, meows => 0 }, $class;
}
sub name { $_[0]->{name} }
sub meow {
my $self = shift;
$self->{meows}++;
return "Meow!";
}
sub meow_count { $_[0]->{meows} }
1;
END
# Create a new Cat object
my $whiskers = $p5.call('Cat::new', 'Cat', 'Whiskers');
# Call methods on the object
say qq:s"My cat is named {$p5.call('Cat::name', $whiskers)}";
# Make Whiskers meow
say qq:s"{$p5.call('Cat::name', $whiskers)} says: {$p5.call('Cat::meow', $whiskers)}";
# Check how many times Whiskers has meowed
say qq:s"Whiskers has meowed {$p5.call('Cat::meow_count', $whiskers)} time(s)";
# Make Whiskers meow again
$p5.call('Cat::meow', $whiskers);
say qq:s"Now Whiskers has meowed {$p5.call('Cat::meow_count', $whiskers)} time(s)";
This example shows how seamlessly you can work with Perl 5 objects in your Raku code.
Wrapping Up
Inline::Perl5 is like a trusty bridge between the familiar shores of Perl 5 and the exciting new lands of Raku. As you grow more comfortable with Raku, try to gradually replace Perl 5 code with native Raku solutions. It’s all part of the learning journey!
Happy coding, and may your scripts be as agile as a cat chasing a laser pointer! 🐱🦋
WWW: 🐪 perl.gg - 🧙♂️ clc.onl - 💾 GitHub - 🏙️ Neocities
Copyright ©️ 2024 raku.gg